Read From a Specific Csv Row Python
Summary: in this tutorial, yous'll learn how to read a CSV file in Python using the built-in csv
module.
What is a CSV file
CSV stands for comma-separated values. A CSV file is a delimited text file that uses a comma to split values.
A CSV file consists of one or more lines. Each line is a data record. And each data record consists of ane or more values separated by commas. In improver, all the lines of a CSV file take the same number of values.
Typically, you use a CSV file to store tabular data in apparently text. The CSV file format is quite popular and supported by many software applications such as Microsoft Excel and Google Spreadsheet.
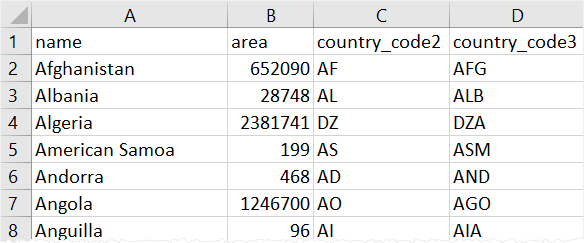
Reading a csv file in Python
To read a CSV file in Python, you follow these steps:
First, import the csv module:
import csv
Code linguistic communication: Python ( python )
Second, open the CSV file using the congenital-in open() function in the read mode:
f = open('path/to/csv_file')
Lawmaking language: Python ( python )
If the CSV contains UTF8 characters, you need to specify the encoding like this:
f = open('path/to/csv_file', encoding='UTF8')
Lawmaking language: Python ( python )
3rd, pass the file object (f
) to the reader()
function of the csv
module. The reader()
function returns a csv reader object:
Lawmaking language: Python ( python )
csv_reader = csv.reader(f)
The csv_reader
is an iterable object of lines from the CSV file. Therefore, you lot can iterate over the lines of the CSV file using a for
loop:
for line in csv_reader: print(line)
Code language: Python ( python )
Each line is a listing of values. To access each value, yous utilize the square bracket annotation []
. The get-go value has an alphabetize of 0. The second value has an alphabetize of 1, and then on.
For example, the following accesses the first value of a particular line:
line[0]
Code linguistic communication: Python ( python )
Finally, ever shut the file once y'all're no longer access it by calling the close()
method of the file object:
Lawmaking language: Python ( python )
f.shut()
It'll be easier to use the with
statement so that yous don't demand to explicitly call the close()
method.
The post-obit illustrates all the steps for reading a CSV file:
import csv with open('path/to/csv_file', 'r') as f: csv_reader = csv.reader(f) for line in csv_reader: # process each line print(line)
Code language: Python ( python )
Reading a CSV file examples
We'll utilise the country.csv
file that contains country information including name, surface area, 2-letter country code, 3-letter country lawmaking:
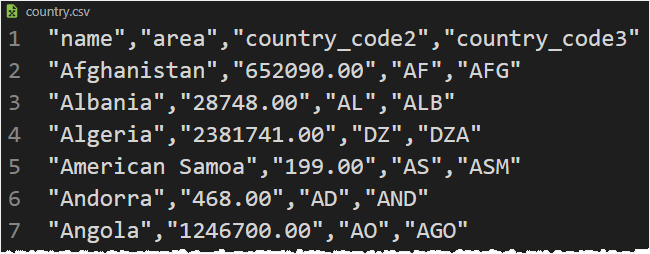
Download state.csv file
The post-obit shows how to read the country.csv
file and display each line to the screen:
import csv with open('state.csv', encoding="utf8") as f: csv_reader = csv.reader(f) for line in csv_reader: print(line)
Code language: Python ( python )
Output:
['proper noun', 'expanse', 'country_code2', 'country_code3'] ['Afghanistan', '652090.00', 'AF', 'AFG'] ['Albania', '28748.00', 'AL', 'ALB'] ['Algeria', '2381741.00', 'DZ', 'DZA'] ['American Samoa', '199.00', 'Every bit', 'ASM'] ...
Lawmaking language: Python ( python )
The country.csv
has the first line equally the header. To separate the header and data, you utilize the enumerate()
function to get the index of each line:
import csv with open up('land.csv', encoding="utf8") as f: csv_reader = csv.reader(f) for line_no, line in enumerate(csv_reader, 1): if line_no == 1: impress('Header:') impress(line) # header print('Data:') else: print(line) # information
Code language: Python ( python )
In this example, nosotros use the enumerate()
function and specify the alphabetize of the first line every bit 1.
Inside the loop, if the line_no is is 1, the line is the header. Otherwise, it's a data line.
Another way to skip the header is to use the adjacent()
function. The next()
function forwards to the reader to the next line. For example:
import csv with open('state.csv', encoding="utf8") as f: csv_reader = csv.reader(f) # skip the commencement row side by side(csv_reader) # prove the information for line in csv_reader: print(line)
Code language: Python ( python )
The post-obit reads the state.csv
file and calculate the total areas of all countries:
import csv total_area = 0 # calculate the total surface area of all countries with open('country.csv', encoding="utf8") as f: csv_reader = csv.reader(f) # skip the header next(csv_reader) # calculate total for line in csv_reader: total_area += float(line[i]) impress(total_area)
Code language: Python ( python )
Output:
148956306.nine
Lawmaking language: Python ( python )
Reading a CSV file using the DictReader class
When y'all apply the csv.reader()
role, you can access values of the CSV file using the bracket notation such as line[0]
, line[one]
, and then on. Notwithstanding, using the csv.reader()
function has two principal limitations:
- Kickoff, the style to access the values from the CSV file is non so obvious. For example, the
line[0]
implicitly means the land name. It would exist more expressive if you can access the land name likeline['country_name']
. - 2nd, when the gild of columns from the CSV file is changed or new columns are added, you need to change the code to become the right data.
This is where the DictReader
class comes into play. The DictReader form also comes from the csv
module.
The DictReader
class allows you to create an object like a regular CSV reader. But it maps the data of each line to a dictionary (dict
) whose keys are specified by the values of the starting time line.
By using the DictReader
class, yous tin access values in the land.csv
file similar line['name']
, line['area']
, line['country_code2']
, and line['country_code3']
.
The following example uses the DictReader
class to read the country.csv
file:
import csv with open up('country.csv', encoding="utf8") as f: csv_reader = csv.DictReader(f) # skip the header next(csv_reader) # prove the data for line in csv_reader: print(f"The area of {line['proper noun']} is {line['area']} km2")
Code language: Python ( python )
Output:
The area of Afghanistan is 652090.00 km2 The area of Albania is 28748.00 km2 The area of Algeria is 2381741.00 km2 ...
Code language: Python ( python )
If you want to accept different field names other than the ones specified in the commencement line, you can explicitly specify them by passing a list of field names to the DictReader()
constructor similar this:
import csv fieldnames = ['country_name', 'area', 'code2', 'code3'] with open('land.csv', encoding="utf8") as f: csv_reader = csv.DictReader(f, fieldnames) adjacent(csv_reader) for line in csv_reader: print(f"The surface area of {line['country_name']} is {line['surface area']} km2")
Code language: Python ( python )
In this example, instead of using values from the first line as the field names, we explicitly pass a list of field names to the DictReader
constructor.
Summary
- Use
csv.reader()
office orcsv.DictReader
grade to read data from a CSV file.
Did you find this tutorial helpful ?
morrisonnetionster.blogspot.com
Source: https://www.pythontutorial.net/python-basics/python-read-csv-file/
0 Response to "Read From a Specific Csv Row Python"
Post a Comment